How To Create a Button in VBA Excel
Adding buttons to Excel worksheets improve user interaction and automation. This guide will walk you through the process of adding and customizing buttons using VBA, making your Excel applications more user-friendly and beautiful.
Table of Contents
Methods to Add Buttons in Excel
Method 1: Using the Developer Tab (Manual)
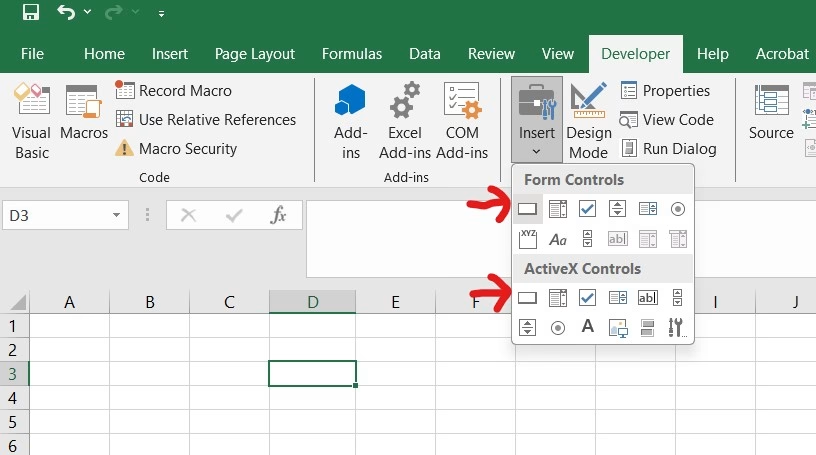
- Access the Developer tab in Excel
- Click ‘Insert’ in the Controls group
- Choose either Form Control or ActiveX Control button
- Draw the button on your worksheet
- Assign a macro to the button
Method 2: Using VBA Code (Programmatic)
If you would like to add a button to your sheet programmatically, here’s some sample code that will do so. To access the VBA editor, Click on the Developer tab and then on VBA.
Sub HelloWorld()
MsgBox ("Hello World!")
End Sub
Sub AddButton()
Dim MyButton As Button
Set MyButton = ActiveSheet.Buttons.Add(100, 100, 100, 30) 'the left, top, width and height
With MyButton
.Name = "MyButton"
.OnAction = "HelloWorld"
.Caption = "Our HelloWorld"
End With
End Sub
In this example, we create two macros or sub-routines: The HelloWorld() sub-routine and the AddButton() sub-routine. You can replace the HelloWorld() sub-routine with whatever actual macro that you want to run when the button is clicked.
Button Properties
These are some of the button properties that you can adjust in Excel VBA to fit your needs.
- Position: Use left and top parameters to set location
- Size: Adjust width and height to fit your needs
- Caption: Customize the text that appears on the button
- Name: Give unique names for easy reference in your code
- OnAction: Specify which macro to run when clicked
Best Practice When Adding Buttons With VBA to Excel Sheets
When adding buttons to Excel sheets programmatically, it is best practice to use error handling and to disable screen updating while the button is being added. Here’s how to do so.
- Error Handling:
Sub DeleteButton()
On Error Resume Next
ActiveSheet.Buttons("MyCustomButton").Delete
On Error GoTo 0
End Sub
Screen Updates:
Application.ScreenUpdating = False
' Your button code here
Application.ScreenUpdating = True
Troubleshooting Tips When Adding Buttons With VBA
You might encounter some issues when adding buttons with VBA. Here are some troubleshooting tips:
- Ensure the Developer tab is enabled
- Check macro security settings
- Verify that the assigned macro exists
- Test button functionality in different Excel versions
Customize Button Appearance in Excel Using VBA
While Excel buttons are primarily functional elements, you can customize their appearance to create a more polished and professional-looking interface. Let’s explore the different ways to customize button appearance using VBA.
Form Control Buttons
Form Control buttons have limited direct customization options. If you’d like to customize the transparency or color of your button, an ActiveX button would work better. It does have some limitations which we’ll mention later.
ActiveX Button Customization
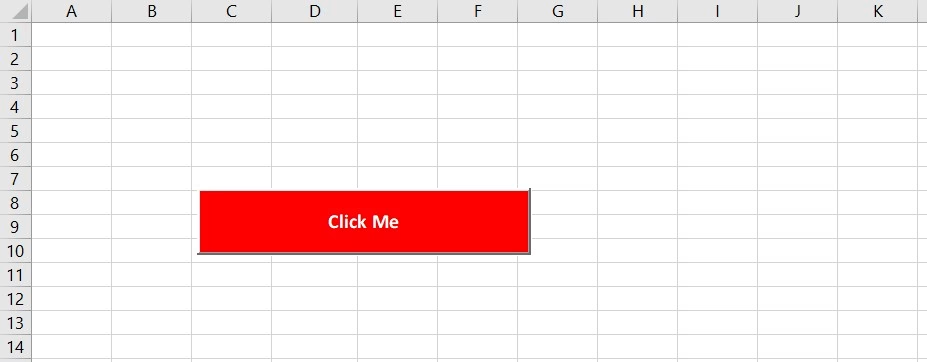
ActiveX buttons offer more direct customization options. The above image is an example of an ActiveX button that was added and then had its color changed using VBA.
Sub AddAndCustomizeActiveXButton()
Dim btn As OLEObject
'Delete existing button if it exists
On Error Resume Next
ActiveSheet.OLEObjects("CommandButton1").Delete
On Error GoTo 0
'Add new ActiveX button
Set btn = ActiveSheet.OLEObjects.Add( _
ClassType:="Forms.CommandButton.1", _
Link:=False, _
DisplayAsIcon:=False, _
Left:=100, _
Top:=100, _
Width:=200, _
Height:=40)
'Customize the button
With btn.Object
.BackColor = RGB(255, 0, 0) ' Red background
.ForeColor = RGB(255, 255, 255) ' White text
.Font.Size = 12
.Font.Bold = True
.Caption = "Click Me"
End With
End Sub
Creative Solutions for Button Styling in Excel
Using Shapes as Buttons in Excel
You aren’t limited to using the Form Control and ActiveX buttons. Excel also allows you to use shapes and even images as buttons. To do so, you assign the ‘On Action” property of the shape or image to a macro. So, when you click the shape, the macro that you assigned will be run.
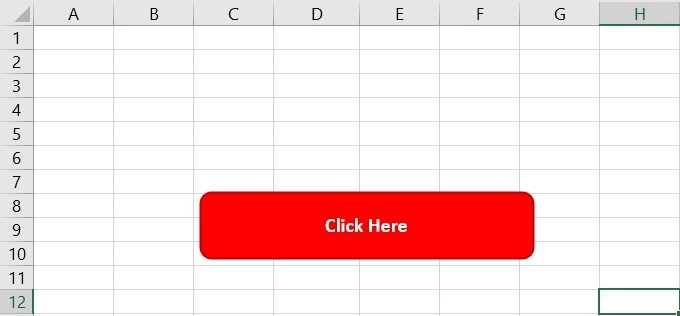
Here is the code to generate a button with rounded edges by using shapes instead. Replace the ‘On Action’ property with the macro that you want to run.
Sub CreateCustomShape()
Dim ws As Worksheet
Dim shp As Shape
Set ws = ActiveSheet
' Add a rounded rectangle shape
' Parameters: Type, Left, Top, Width, Height
Set shp = ws.Shapes.AddShape(msoShapeRoundedRectangle, 100, 100, 200, 40)
With shp
' Set the fill color to red
.Fill.ForeColor.RGB = RGB(255, 0, 0)
' Set border properties
With .Line
.ForeColor.RGB = RGB(200, 0, 0) ' Darker red border
.Weight = 1.5 ' Border thickness
End With
' Add and format text
With .TextFrame
.Characters.Text = "Click Here"
.Characters.Font.Color = RGB(255, 255, 255) ' White text
.Characters.Font.Size = 12
.Characters.Font.Bold = True
.HorizontalAlignment = xlCenter
.VerticalAlignment = xlCenter
.MarginLeft = 5
.MarginRight = 5
End With
' Assign macro (optional)
.OnAction = "YourMacroName"
End With
End Sub
Best Practices When Adding Buttons
When adding buttons to your Excel sheet, keep the following in mind:
- Consistency: Maintain consistent styling across all buttons
- Contrast: Ensure text is readable against the button background
- Size: Make buttons large enough to be easily clickable
- Feedback: Consider adding hover or click effects for better user experience
Limitations of Various Button Types in Excel
As we’ve covered in this article, there are three options when it comes to adding buttons in Excel. Form Control buttons are the most basic, ActiveX provide more customization options and with shapes / images, you can get professionally customized buttons in your spreadsheet.
They all have their own limitations, though:
- Form Control buttons have restricted customization options
- ActiveX controls only work on Windows
- Some customizations may not be visible in different Excel versions
Troubleshooting Tips if Your Excel Buttons Aren’t Working
These are some basic troubleshooting steps you might want to follow if your buttons aren’t appearing or working the way you want them to.
- If colors aren’t showing:
- Check if ActiveX controls are enabled
- Verify the control type (Form vs ActiveX)
- If shapes aren’t working as buttons:
- Ensure macros are enabled
- Check the OnAction property assignment
Final Thoughts on Creating Buttons in VBA Excel
Buttons are a common way to make it more convenient for users to run macros. You can add them manually or use VBA to add them programmatically. If you would like to customize your buttons, consider using ActiveX buttons or shapes but keep in mind their limitations around compatibility.